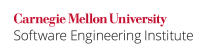
Scope minimization helps developers avoid common programming errors, improves code readability by connecting the declaration and actual use of a variable, and improves maintainability because unused variables are more easily detected and removed. It may also allow objects to be recovered by the garbage collector more quickly, and it prevents violations of DCL51-J. Do not shadow or obscure identifiers in subscopes.
Noncompliant Code Example
This noncompliant code example shows a variable that is declared outside the for
loop.
public class Scope { public static void main(String[] args) { int i = 0; for (i = 0; i < 10; i++) { // Do operations } } }
This code is noncompliant because, even though variable i
is not intentionally used outside the for
loop, it is declared in method scope. One of the few scenarios where variable i
would need to be declared in method scope is when the loop contains a break statement and the value of i
must be inspected after conclusion of the loop.
Compliant Solution
Minimize the scope of variables where possible. For example, declare loop indices within the for
statement:
public class Scope { public static void main(String[] args) { for (int i = 0; i < 10; i++) { // Contains declaration // Do operations } } }
Noncompliant Code Example
This noncompliant code example shows a variable count
that is declared outside the counter
method, although the variable is not used outside the counter
method.
public class Foo { private int count; private static final int MAX_COUNT = 10; public void counter() { count = 0; while (condition()) { /* ... */ if (count++ > MAX_COUNT) { return; } } } private boolean condition() {/* ... *} // No other method references count // but several other methods reference MAX_COUNT }
Compliant Solution
In this compliant solution, the count
field is declared local to the counter()
method:
public class Foo { private static final int MAX_COUNT = 10; public void counter() { int count = 0; while (condition()) { /* ... */ if (count++ > MAX_COUNT) { return; } } } private boolean condition() {/* ... */} // No other method references count // but several other methods reference MAX_COUNT }
Applicability
Detecting local variables that are declared in a larger scope than is required by the code as written is straightforward and can eliminate the possibility of false positives.
Detecting multiple for
statements that use the same index variable is straightforward; it produces false positives in the unusual case where the value of the index variable is intended to persist between loops.
Bibliography
Item 29, "Minimize the Scope of Local Variables" | |
[JLS 2013] |
3 Comments
Dhruv Mohindra
count
variable would need to be redefined in the new context."James Ahlborn
In the intro section you might also want to mention that incorrect scoping can cause memory "leaks" (holding on to no longer useful objects longer than necessary).
Fred Long
Done.