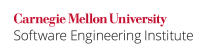
Enumerations in C++ come in two forms: scoped enumerations, where the underlying type is fixed, and unscoped enumerations where the underlying type is not fixed. The range of values that can be represented by either form of enumeration may include enumerator values not specified by the enumeration itself. The range of valid enumeration values for an enumeration type is defined by the C++ Standard, [dcl.enum], paragraph 8 [ISO/IEC 14882-2014]:
For an enumeration whose underlying type is fixed, the values of the enumeration are the values of the underlying type. Otherwise, for an enumeration where emin is the smallest enumerator and emax is the largest, the values of the enumeration are the values in the range bmin to bmax, defined as follows: Let K be
1
for a two’s complement representation and0
for a one’s complement or sign-magnitude representation. bmax is the smallest value greater than or equal to max(|emin| − K, |emax|) and equal to 2M − 1, where M is a non-negative integer. bmin is zero if emin is non-negative and −(bmax + K) otherwise. The size of the smallest bit-field large enough to hold all the values of the enumeration type is max(M, 1) if bmin is zero and M + 1 otherwise. It is possible to define an enumeration that has values not defined by any of its enumerators. If the enumerator-list is empty, the values of the enumeration are as if the enumeration had a single enumerator with value0
.
According to the C++ Standard, [expr.static.cast], paragraph 10,
A value of integral or enumeration type can be explicitly converted to an enumeration type. The value is unchanged if the original value is within the range of the enumeration values (7.2). Otherwise, the resulting value is unspecified (and might not be in that range). A value of floating-point type can also be explicitly converted to an enumeration type. The resulting value is the same as converting the original value to the underlying type of the enumeration (4.9), and subsequently to the enumeration type.
To avoid operating on unspecified values, the arithmetic value being cast must be within the range of values the enumeration can represent. When checking for out-of-range values dynamically, it must be performed prior to the cast expression.
Noncompliant Code Example (Bounds Checking)
This noncompliant code example attempts to check whether a given value is within the range of acceptable enumeration values. However, it is doing so after casting to the enumeration type, which may not be able to represent the given integer value. On a two's complement system, the valid range of values that can be represented by enum_type
are: [0..3], so if a value outside of that range were passed to f()
, the cast to enum_type
would result in an unspecified value, and using that value within the if
statement results in unspecified behavior.
enum enum_type { E_A, E_B, E_C }; void f(int int_var) { enum_type enum_var = static_cast<enum_type>(int_var); if (enum_var < E_A || enum_var > E_C) { // Handle error } }
Compliant Solution (Bounds Checking)
This compliant solution checks that the value is within the range of acceptable enumeration values before the conversion to guarantee there is no unspecified result. It further restricts the converted value to one for which there is a specific enumerator value.
enum enum_type { E_A, E_B, E_C }; void f(int int_var) { if (int_var < E_A || int_var > E_C) { // Handle error } enum_type enum_var = static_cast<enum_type>(int_var); }
Compliant Solution (Scoped Enumeration)
This compliant solution uses a scoped enumeration, which has a fixed underlying type of type int
by default, allowing any value from the parameter to be converted into a valid enumeration value. It does not further restrict the converted value to one for which there is a specific enumerator value, but could do so by using the previous compliant solution.
enum class enum_type { E_A, E_B, E_C }; void f(int int_var) { enum_type enum_var = static_cast<enum_type>(int_var); }
Risk Assessment
Unexpected behavior can lead to a buffer overflow and the execution of arbitrary code by an attacker. This is most likely if the program in one case checks the value correctly and then fails to do so later. Such a situation could allow an attacker to avoid verification of a buffer's length, and so on.
Automated detection should be possible for most cases, but it might not be able to guarantee if the value in range.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
INT30-CPP | Medium | Unlikely | Medium | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Bibliography
[ISO/IEC 14882-2014] | 7.2, "Enumeration Declarations" |
[Becker 2009] | Section 7.2, "Enumeration declarations" |